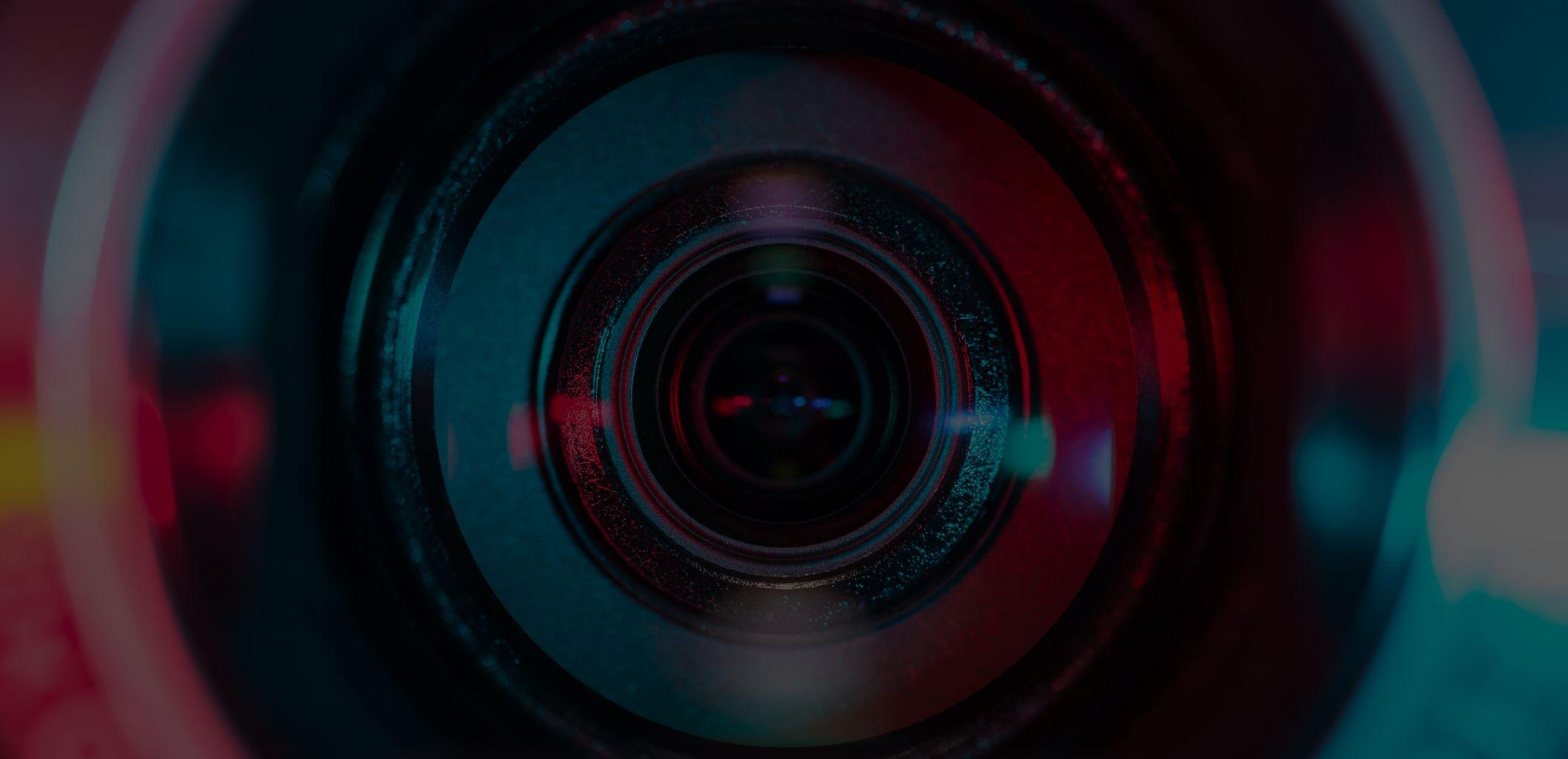
How to Integrate Twilio Segment with Fingerprint on a Next.js Site
Enhance the accuracy of your Segment user profile dataset by implementing Fingerprint's accurate device-level tracking.
Twilio Segment takes the general benefits of website segmentation and supercharges them, especially for B2B companies. Here's how it specifically benefits marketing teams:
Deep understanding of the B2B buying journey to decipher complex sales cycles and enable ABM (Account-based Marketing).
Real-time personalization at scale by enabling marketers to craft detailed personas based on firmographic data, website behavior, and other attributes.
Marketing Automation using automated workflows based on website activity.
Attribution modelling for data-driven budget allocation and improving campaign ROI.
However, Segment is only as powerful as it is accurate.
Enter: Fingerprint
Fingerprint is a device intelligence platform (built on the Open Source FingerprintJS) that helps businesses identify and understand their online visitors by creating unique fingerprints for each device, even when cookies are disabled or users browse in private modes.
Why pair these two together?
While Segment excels at capturing and organizing customer data, it may not be the most accurate solution for granular user identification. Although each request does include identifying properties, these values might not always include the most precise view of individual users. FingerprintJS complements Segment by offering superior device fingerprinting capabilities, enabling businesses to gain a more accurate and comprehensive understanding of their online visitors for even more refined segmentation.
Implementation
There are many ways to include both Segment and Fingerprint on your site, but this guide will be using NPM packages running on Next.js.
Segment Installation
Installing Segment to your site is a bit more hands on compared to let’s say Google Analytics and will require your team to outline all of the requirements for data collection. It roughly breaks down into these 4 calls to generate quality data.
Page Calls - When a user loads a page
Identify Calls - Ties a user to actions
Track Calls - Actions performed by a user
Group Calls - Associate users with a defined group
There are other calls that Segment offers but these will be the most impactful.
That being said, let’s get into the steps to just get Page Calls running using the Analytics.js frontend method using Next.js.
Step One
Create your Segment account and follow default set up procedures.
Step Two
In this step, we are only going to implement the most basic Page Call features. You will need to create a source, then either install the snippet directly in the <head> of your site or include their NPM package @segment/analytics-next and use the imported load methods. Be sure to include the sources Segment Write Key to connect correctly. This will need to be run on every new page render to return data to Segment.
Via NPM
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15
// Import import { AnalyticsBrowser } from '@segment/analytics-next' const Tracking = () => { // Trigger the load instance of Segment in your app const analytics = AnalyticsBrowser.load({ writeKey: proccess.env.NEXT_PUBLIC_SEGMENT_WRITE_KEY }) // Hook to handle states useEffect(() => { // Send the data to Segment analytics.page(); }, []); } export default Tracking;
Step Three
Download the browser extension Segment Event Tracker to visualize and debug live Segment calls an your site to cross compare sent and stored data.
This is your most basic installation of Segment and can only become more refined from here with the different calls available.
FingerprintJS Installation
Installing FingerprintJS is similar to Segment as it requires an account, provides a vanilla JS approach with alternative NPM package, and needs to be initialized on all page views. There is less configuration on base installation as it has a more plug and play approach.
Step One
Create your Fingerprint account and follow default set up procedures.
Step Two
Within the Fingerprint dashboard, navigate to API Keys then generate a new Public Key and leave the default settings for now. Be sure to name it appropriately as it helps with dashboard hygiene.
Step Three
Luckily they make installation as easy as possible. Navigate to the Get Started tab which will provide a step by step guide to configure a platform, select the framework to install with, then verify. Since this is for NextJS, install the NPM package, wrap the component you want to track with the context provider, and initialize the hook where needed.
For this example, we wrap the entire content component and call Fingerprint on every page.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17
// pages/_app.tsx import { Tracking } from '../components'; import { FpjsProvider } from '@fingerprintjs/fingerprintjs-pro-react' export default function MyApp({Component, pageProps}: AppProps) { return ( <FpjsProvider loadOptions={{ apiKey: process.env.NEXT_PUBLIC_FINGERPRINTJS_API_KEY ?? '', }} > <Component {...pageProps} /> <Tracking /> </FpjsProvider> ) }
Then we trigger the track calls in our Track component.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16
// components/Tracking.tsx import { useVisitorData } from '@fingerprintjs/fingerprintjs-pro-react'; const Tracking = () => { // Initilize tracking const { isLoading, error } = useVisitorData( { extendedResult: true }, { immediate: true } ); // Hook to handle states useEffect(() => { if (!isLoading && error) { console.error('Error fetching visitor data:', error); } }, [isLoading, error]); } export default Tracking;
Fingerprint Integration into Segment
Fingerprint provides a solution in their docs which converts the installed instance into a Source as seen here. This approach is achieved through some configuration on Segment to accept incoming calls to from Fingerprint and will mutate the data with Fingerprint data before sending it to the connected destinations. Data collected in this approach will not alter and Segment data. Alternatively, we can go off script and directly mutate Segment data on the page before sending it.
Fingerprint Provided Approach
Fingerprint has included a full guide on connecting to Segment via webhook. This will send a post request to a Segment Source Function which will mutate the Segment data and forward it to the destination. Below is an abridged version and the full implementation can be found here.
Step One
In Segment, create a new Segment Function, download the latest Fingerprint Segment source function, and apply the downloaded to the function.
Step Two
Create a Segment Source connected to the Fingerprint Source function to intertwine the data stored in Segment and data sent from Fingerprint. In the source overview page, retrieve the webhook URL for step three.
Step Three
In Fingerprint, create a new webhook and add the url for the source function retrieved in step two.
Step Four
Within your site, you will need to include additional configurations when making the initial call to Fingerprint. This skipIntegration flag enables calls to Segment Source Function to validate and determine which data and whether additional fields/overrides are to be included.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25
// components/Tracking.tsx import { useVisitorData } from '@fingerprintjs/fingerprintjs-pro-react'; const Tracking = () => { // Initilize tracking const { isLoading, error } = useVisitorData( { extendedResult: true, tag: { integrations: { segment: { skipIntegration: false, }, }, }, }, { immediate: true } ); // Hook to handle states useEffect(() => { if (!isLoading && error) { console.error('Error fetching visitor data:', error); } }, [isLoading, error]); } export default Tracking;
This implementation will include the data highlighted in their integration doc and does not include any additional custom fields.
This approach does allow you to pass modified data to Segment destinations but are not included in the dashboard as the calls and separated from the core data set.
Alternative Direct Segment Mutation Approach
Since the data is only sent to it’s destinations after hitting the Fingerprint webhook and Segment Source Function, you will not be benefiting from the Segment dashboard being enhanced by Fringerprint data. So, this approach will be directly mutating Segment data with Fingerprint before sending the request from the website. There is more coding involved with this so some examples have been included in the steps.
Step One
Access data provided by Fingerprint when invoking the hook for the first time.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25
// components/Tracking.tsx import { useVisitorData } from '@fingerprintjs/fingerprintjs-pro-react'; const Tracking = () => { // Initilize tracking const { isLoading, error } = useVisitorData( { extendedResult: true, tag: { integrations: { segment: { skipIntegration: false, }, }, }, }, { immediate: true } ); // Hook to handle states useEffect(() => { if (!isLoading && error) { console.error('Error fetching visitor data:', error); } }, [isLoading, error]); } export default Tracking;
Step 2
Use the addSourceMiddleware method from the analytics object to mutate all outgoing requests to Segment. You will need to wait and make sure that Fingerprint data has loaded before setting up the middleware callback.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19
// Hook to handle states useEffect(() => { if (!isLoading && error) { console.error('Error fetching visitor data:', error); } // If Fingerprint is done loading, data has been returned, and there is no error if(!isLoading && data && !error) { analytics.addSourceMiddleware(({ payload, next }) => { const { obj } = payload; payload.obj = { ...payload.obj, // Override anonymousId from Segment with visitorId from Fingerprint anonymousId: data.visitorId }; } next(payload); }); } }, [isLoading, error, data]);
Note: This approach will alter the data stored in Segment which can become compromised if you were to ever remove Fingerprint as user values will return to default.
Conclusion
Fingerprint is a complimentary addition to enhancing the dataset collected by Segment creating more accurate and valuable user profiling.
Implementation is up to you when it comes to how you would like to store and forward the data.